Not sure if this is completely correct and robust enough for what you need, but it works. At least for H and O.
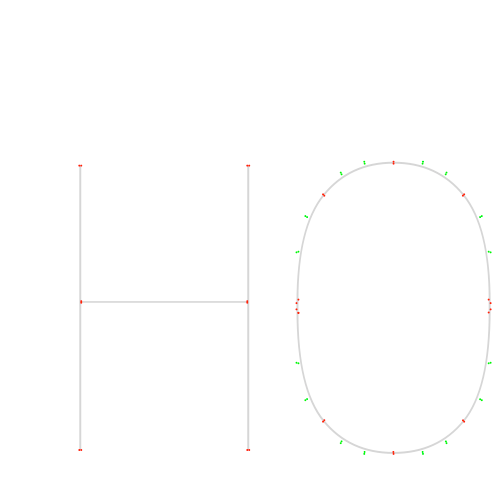
# Set up canvas
canvas_width = 500
canvas_height = 500
txt = "HO"
variable_font_path = "RobotoFlex-VariableFont_GRAD,XOPQ,XTRA,YOPQ,YTAS,YTDE,YTFI,YTLC,YTUC,opsz,slnt,wdth,wght.ttf"
font_size = 400
point_size = 2
# Neue Seite erstellen und Hintergrund setzen
newPage(canvas_width, canvas_height)
fill(1) # Weißer Hintergrund
rect(0, 0, canvas_width, canvas_height)
fill(0) # Schwarzer Text
# Schriftart setzen und prüfen, ob sie korrekt geladen wird
font(variable_font_path)
fontSize(font_size)
# Alle Achsen der aktuellen Schriftart auflisten
variations = listFontVariations()
# for axis, data in variations.items():
# print(f"Axis: {axis}, Data: {data}")
# Eine Variation der aktuellen Schriftart auswählen
if 'wght' in variations:
fontVariations(wght=500)
# Create a FormattedString with the text and variable font
formattedString = FormattedString(txt, font=variable_font_path, fontSize=font_size)
# Get the Bézier path of the text using FormattedString
path = BezierPath()
path.text(formattedString, (50, 50))
with savedState():
fill(.8)
drawPath(path)
# Initialize lists to hold on-curve and off-curve points
on_curve_points = []
off_curve_points = []
# Iterate through contours and segments to extract points
output = ""
for contour in path.contours:
# moveTo
x, y = contour[0][0]
on_curve_points.append((x, y))
for segment in contour[1:]:
if len(segment) == 1:
# lineTo
pt = segment[0]
on_curve_points.append((pt[0], pt[1]))
if len(segment) == 3:
# curveTo
cp1 = segment[0]
cp2 = segment[1]
pt = segment[2]
off_curve_points.append((cp1[0], cp1[1]))
off_curve_points.append((cp2[0], cp2[1]))
on_curve_points.append((pt[0], pt[1]))
# Draw on-curve points
fill(1, 0, 0) # Rot für on-curve Punkte
stroke(None)
for (x, y) in on_curve_points:
print("Drawing on-curve point at:", x, y)
oval(x - point_size / 2, y - point_size / 2, point_size, point_size)
# Draw off-curve points
fill(0, 1, 0) # Grün für off-curve Punkte
stroke(None)
for (x, y) in off_curve_points:
print("Drawing off-curve point at:", x, y)
oval(x - point_size / 2, y - point_size / 2, point_size, point_size)
# Save the image
saveImage("H_with_anchor_points.png")
# Ausgabe der Koordinaten
print(output)