hello @mït,
I think I understand what you mean – it’s not so easy because, once a piece of text is drawn into the page, you can’t really ‘search’ for it anymore.
it can be done if you lay out the text yourself: word by word, line by line, page by page. the example script below is based on this one.
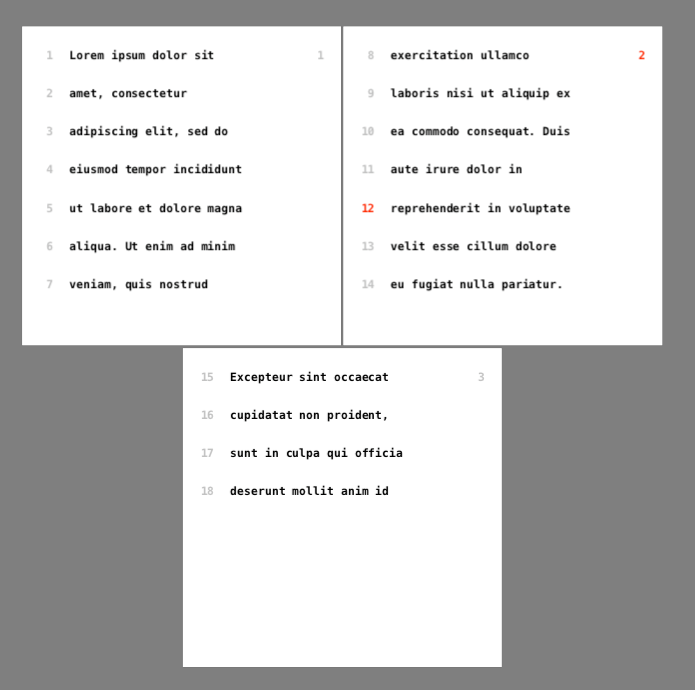
TXT = "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum."
FONT = 'Menlo-Bold'
FONTSIZE = 36
LINEHEIGHT = 120
WIDTH = 570
X, Y = 150, 900
HIGHLIGHT = 'voluptate'
PAGEHIGHLIGHT = None
# start with an empty string, add words one by one
T = FormattedString(font=FONT, fontSize=FONTSIZE, lineHeight=FONTSIZE*1.2)
# set text properties globally - needed for correct measurements with `textSize`
font(FONT)
fontSize(FONTSIZE)
# variables to keep track of the current line
lineLength = 0
lineNumber = 1
lineY = Y
# iterate over words in text
for word in TXT.split():
# if word fits in the current line, add word to line
if lineLength + textSize(word)[0] <= WIDTH:
T += word + ' '
lineLength += textSize(word + ' ')[0]
# word does not fit in current line, make a new line
else:
# if next line does not fit in current page, start a new page
if lineY - LINEHEIGHT < 0:
newPage()
font(FONT)
fontSize(FONTSIZE)
lineY = Y
# draw the current line
text(T, (X, lineY))
# check if highlight word was in this line and page
if HIGHLIGHT in str(T):
PAGEHIGHLIGHT = pageCount()
color = (1, 0, 0)
else:
color = (0.7,)
fill(*color)
text(str(lineNumber), (X-50, lineY), align='right')
# make a fresh new line, add word to line
T = FormattedString(font=FONT, fontSize=FONTSIZE, lineHeight=FONTSIZE*1.2)
T += word + ' '
# update variables for new line
lineLength = textSize(word + ' ')[0]
lineNumber += 1
lineY -= LINEHEIGHT
# draw page numbers, check if highlight word in page
for i, page in enumerate(pages()):
with page:
color = (1, 0, 0) if (i+1) == PAGEHIGHLIGHT else (0.7,)
fill(*color)
text(str(i+1), (width()-50, Y), align='right')
hope this makes sense!